
In the world of object-oriented programming, two fundamental concepts play a crucial role in designing efficient and flexible code: inheritance and polymorphism. These concepts not only enhance code readability but also contribute to the reusability of code, making it easier to maintain and scale. In this article, we will explore the similarities between inheritance and polymorphism, shedding light on how they complement each other in creating robust software solutions.
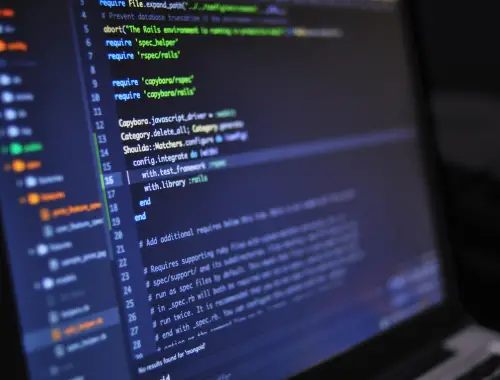
Conceptual Foundation:
- Both inheritance and polymorphism are pillars of object-oriented programming (OOP) and share the foundational principle of code organization through the use of classes and objects.
Code Reusability:
- Inheritance allows the creation of a new class (subclass) by inheriting the properties and behaviors of an existing class (superclass). This promotes code reusability, as common attributes and methods need not be rewritten in each subclass.
- Polymorphism, on the other hand, enables objects of different classes to be treated as objects of a common base class. This flexibility in handling different objects with a shared interface enhances code reuse and simplifies system expansion.
Abstraction:
- Inheritance and polymorphism contribute to abstraction by allowing developers to model real-world relationships and entities in a more intuitive and modular way. Abstraction enables the extraction of essential features while hiding unnecessary details, enhancing the clarity and maintainability of the code.
Method Overriding:
- Both concepts involve the ability to override methods. In inheritance, a subclass can provide a specific implementation for a method already defined in its superclass. In polymorphism, method overriding allows different classes to implement their own version of a method declared in a common interface or base class.
Dynamic Binding:
- Polymorphism, particularly runtime polymorphism achieved through method overriding, involves dynamic binding. This means that the decision of which method to invoke is made during runtime, promoting flexibility and adaptability in the code.
Here’s a comparison table highlighting the key similarities between Inheritance and Polymorphism
Aspect | Inheritance | Polymorphism |
---|---|---|
Definition | Mechanism inheriting properties from a base class. | Concept allowing objects to be treated uniformly. |
Relationship | Hierarchical (Parent-Child) class relationship. | Objects of different classes treated uniformly. |
Code Reusability | Promotes code reuse by inheriting attributes. | Promotes code reuse by providing a common interface. |
Implementation | Keywords like extends or : public establish. | Utilizes interfaces, abstract classes, and overrides. |
Base Class | Class from which properties are inherited. | Serves as a common interface for polymorphic behavior. |
Type Compatibility | Subclasses compatible with their base class. | Objects treated as instances of their base class. |
Method Overriding | Subclasses can override methods from the base class. | Derived classes provide their implementation. |
Dynamic Binding | Supports dynamic binding for method selection. | Achieves dynamic binding through method overriding. |
Inheritance, by establishing an “is-a” relationship between subclasses and superclasses, contributes to dynamic binding by allowing objects to be treated as instances of their superclass.
Inheritance and polymorphism are interconnected concepts that empower developers to create flexible, scalable, and maintainable code. The shared emphasis on code reusability, abstraction, method overriding, and dynamic binding highlights their synergy in the realm of object-oriented programming. By leveraging these similarities, developers can design software solutions that not only reflect real-world relationships accurately but also adapt seamlessly to evolving requirements.
Q1: What is the main similarity between Inheritance and Polymorphism?
- A: The primary similarity between Inheritance and Polymorphism is that both concepts contribute to code reuse and flexibility in object-oriented programming.
Q2: How do Inheritance and Polymorphism promote code reuse?
- A: Inheritance achieves code reuse by allowing a new class (subclass) to inherit properties and behaviors from an existing class (base class). Polymorphism promotes code reuse by enabling objects of different classes to be treated as objects of a common type, fostering a shared interface.
Q3: Are there specific keywords or mechanisms associated with Inheritance and Polymorphism?
- A: Yes, Inheritance is often implemented using keywords like
extends
(in Java) or: public
(in C++). Polymorphism, on the other hand, is achieved through interfaces, abstract classes, and method overriding.
Q4: How do Inheritance and Polymorphism contribute to code organization?
- A: Inheritance establishes a hierarchical relationship between classes, organizing them in a parent-child structure. Polymorphism introduces a common interface, providing a structured way to interact with objects of different types.
Q5: Can you provide examples of Inheritance and Polymorphism in real-world scenarios?
- A: In a real-world scenario, consider a base class “Vehicle” with subclasses like “Car” and “Motorcycle” (Inheritance). Polymorphism can be seen when various vehicle objects, regardless of their specific type, can be treated uniformly—for example, calling a common method like
start()
on any vehicle object.
Q6: How do Inheritance and Polymorphism enhance flexibility in programming?
- A: Inheritance enhances flexibility by allowing the creation of new classes based on existing ones, facilitating code extension. Polymorphism enhances flexibility by enabling objects to take on multiple forms, allowing for more dynamic and adaptable interactions.
Q7: Can Inheritance and Polymorphism be used together in a program?
- A: Absolutely. In fact, using Inheritance and Polymorphism together is a common practice. Inheritance establishes relationships between classes, and polymorphism ensures that objects can be treated uniformly, irrespective of their specific class.
Q8: Do Inheritance and Polymorphism both support dynamic behavior in a program?
- A: Yes, both Inheritance and Polymorphism contribute to dynamic behavior. Inheritance supports dynamic binding, allowing the selection of methods at runtime, while Polymorphism achieves dynamic behavior through method overriding and late binding.
Q9: How do Inheritance and Polymorphism impact the maintainability of code?
- A: Both Inheritance and Polymorphism enhance code maintainability. Inheritance reduces redundancy by allowing shared attributes and methods to be defined in a common base class. Polymorphism simplifies code by providing a unified interface, making it easier to understand and modify.
Q10: Can you explain how Inheritance and Polymorphism contribute to a more modular code structure?
- A: Inheritance promotes modularity by organizing code into a hierarchy of classes, each responsible for specific functionalities. Polymorphism adds modularity by allowing interchangeable components, as objects of different classes can be used interchangeably where a common interface is expected.
Q11: Are there any potential pitfalls or challenges associated with using Inheritance and Polymorphism?
- A: One challenge with Inheritance is the potential for deep hierarchies, leading to complex relationships. With Polymorphism, careful consideration is needed to ensure that the common interface is well-defined and consistent across different classes to avoid unexpected behavior.
Q12: How do Inheritance and Polymorphism contribute to the concept of “code extensibility”?
- A: Inheritance facilitates code extensibility by allowing the creation of new classes that extend or specialize the behavior of existing classes. Polymorphism adds to code extensibility by enabling the integration of new classes without modifying existing code, as long as they adhere to a common interface.
Q13: Can you provide examples of programming languages that heavily rely on Inheritance and Polymorphism?
- A: Java, C++, and Python are examples of programming languages that heavily utilize Inheritance and Polymorphism. Java and C++ use explicit keywords for these concepts, while Python implements them more implicitly.
Q14: How do Inheritance and Polymorphism contribute to the principle of “code abstraction”?
- A: Inheritance supports code abstraction by allowing the creation of abstract classes with common attributes and methods. Polymorphism adds abstraction by providing a unified interface, abstracting away the specific details of different object types.
Q15: Can you explain how Inheritance and Polymorphism impact the efficiency of software development?
- A: Inheritance and Polymorphism contribute to more efficient software development by promoting code reuse, reducing redundancy, and enhancing the overall structure of the code. This, in turn, leads to faster development cycles and easier maintenance.
Q16: How do Inheritance and Polymorphism contribute to the concept of “object-oriented design principles”?
- A: Inheritance aligns with the “Open/Closed Principle” by allowing for the extension of behavior without modifying existing code. Polymorphism supports the “Liskov Substitution Principle” by enabling the interchangeability of objects of related classes through a common interface.
Q17: Can Inheritance and Polymorphism be used in other programming paradigms, or are they exclusive to object-oriented programming?
- A: While Inheritance and Polymorphism are integral to object-oriented programming, some aspects can be found in other paradigms. In functional programming, for example, polymorphic behavior can be achieved through higher-order functions, and hierarchical structures may be expressed differently.
Q18: How do Inheritance and Polymorphism contribute to the concept of “runtime flexibility”?
- A: Inheritance provides runtime flexibility by allowing the selection of methods at runtime based on the actual type of the object (dynamic binding). Polymorphism adds to runtime flexibility by enabling objects to exhibit different behaviors during program execution, enhancing adaptability.
Q19: In what scenarios would you choose to use Inheritance over Polymorphism, or vice versa?
- A: Inheritance is beneficial when creating a hierarchy of classes with shared attributes and behaviors. Polymorphism is useful when you want objects of different classes to be treated uniformly through a common interface. Often, both are used in conjunction for a comprehensive solution.
Q20: Can Inheritance and Polymorphism be considered best practices in software development, or are there situations where they might be avoided?
- A: Inheritance and Polymorphism are generally considered best practices in object-oriented programming. However, overusing deep hierarchies or creating overly complex polymorphic relationships may lead to maintenance challenges. Careful design and consideration of specific project requirements are essential.
Leave a Reply